We’ll start with an overview of Plaid and then jump start your integration with a step-by-step guide and code samples in Ruby, Python, and Node. If you’d like to follow along (and test it out!), clone the quickstart repository for the complete example apps. You’ll want to sign up for free API keys to get started.
Running the walkthrough app
Running the walkthrough app
git clone https://github.com/plaid/quickstart.git
cd quickstart/node
npm install
# Start the Quickstart with your API keys from the Dashboard
# https://dashboard.plaid.com/team/keys
PLAID_CLIENT_ID='CLIENT_ID' \
PLAID_SECRET='SECRET' \
PLAID_PUBLIC_KEY='PUBLIC_KEY' \
PLAID_ENV='sandbox' \
PLAID_PRODUCTS=auth,transactions \
node index.js
# Go to http://localhost:8000
Running the walkthrough app for the OAuth flow
Some European institutions require an OAuth redirect authentication flow, where the end user is redirected to the bank’s website or mobile app to authenticate. For this flow, you should provide two additional configuration parameters, PLAID_OAUTH_NONCE
and PLAID_OAUTH_REDIRECT_URI
.
You will need to whitelist the PLAID_OAUTH_REDIRECT_URI
for your client ID through the Plaid developer dashboard at https://dashboard.plaid.com/team/api. Additionally, please set PLAID_OAUTH_NONCE
to a unique identifier such as a UUID. The nonce must be at least 16 characters long. For more details, please refer to the OAuth docs.
Running the walkthrough app for the OAuth flow
git clone https://github.com/plaid/quickstart.git
cd quickstart/node
npm install
# Start the Quickstart with your API keys from the Dashboard
# https://dashboard.plaid.com/account/keys
PLAID_CLIENT_ID='CLIENT_ID' \
PLAID_SECRET='SECRET' \
PLAID_PUBLIC_KEY='PUBLIC_KEY' \
PLAID_ENV='sandbox' \
PLAID_PRODUCTS='transactions' \
PLAID_COUNTRY_CODES='GB' \
PLAID_OAUTH_REDIRECT_URI='http://localhost:8000/oauth-response.html'
\
PLAID_OAUTH_NONCE='nice-and-long-nonce' \
node index.js
# Go to http://localhost:8000
Platform Overview
To help you get oriented with Plaid’s API and what it can help you do, let’s start by defining some basics:
API Keys
You have three different API keys
public_key
a non-sensitive, public identifier that is used to initialize Plaid Link
secret
and client_id
private identifiers that are required for accessing any financial data
these should never be shared in client-side code
Though client_id
and public_key
are the same across all environments, you have a unique secret
for each API environment. Use our Sandbox and Development environments to build out and test your integration with simulated and live users, respectively. You’ll move over to our Production environment once you’re ready to go live!
When using our Sandbox environment, use user_good
as the username and pass_good
for the password. See the docs for more.
API environments
sandbox
: Stateful sandbox environment; use test credentials and build out and test your integrationdevelopment
: Test your integration with live credentials; you will need to request access before you can access our Development environmentproduction
: Production API environment; all requests are billed
Item overview
Most API requests interact with an Item
, a set of credentials (map of key value pairs) associated with a financial institution. A single end-user of your application might have accounts at different financial institutions, which means they would have multiple different Item
s.
Your user can have multiple Item
s, or sets of credentials
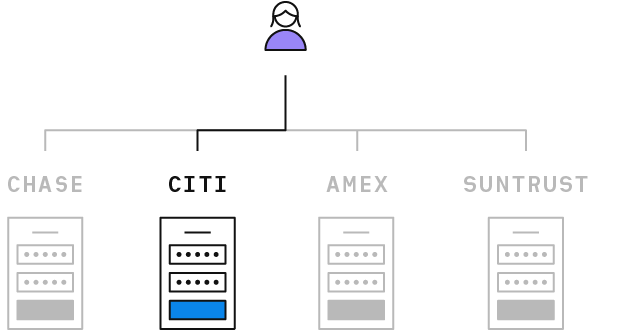
Each Item
can have many associated accounts, which hold information such as balance, name, and account type
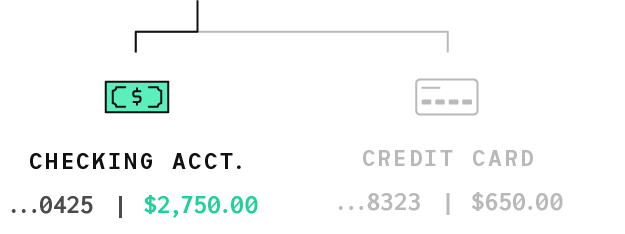
Credit and depository accounts may also have transactions associated with them.
Your users create Item
s through Link, a drop-in module that handles the credential and MFA validation process. Once an Item
is created, Link passes a public_token
that you exchange for an access_token
from your backend app server.
That access_token
and item_id
uniquely identify the Item
. You use the access_token
along with your client_id
and secret
to access products available for an Item
and make changes to it over time.
Read on for more information about each of our products and how you create and access data for an Item
.
Plaid Products
Accessing data
Once you create an Item
, you can then access data—such as transaction data and account and routing numbers—using our API endpoints. You access data for an Item
using the Item
’s access_token
, which is specific to your API keys and cannot be shared or used by any other API keys. By default, an access_token
never expires, but you can rotate it.
You can use the /item/get
endpoint to inspect the status of an Item
and see a list of all available products as well as the ones you’re actively using. You can then call different product endpoints to retrieve data. Due to the complexity of communicating with financial institutions, the initial product data request may take some time.
To integrate an existing architecture with the Plaid API, you might try using a table to store access_token
and item_id
combinations that map to individual users in your system.
Products overview
Auth | Retrieve account and routing numbers for ACH authentication. No micro-deposits required. |
Transactions | Clean transaction data going back as far as 24 months. Transaction data may include context such as geolocation, merchant, and category information. |
Identity | Identity information on file with the bank. Reduce fraud by comparing user-submitted data to validate identity. This product has to be enabled separately in Development and Production. Contact us for more information |
Balance | Check balances in real time to prevent non-sufficient funds fees. |
Income | Verify employment and income information. This product has to be enabled separately in Development and Production. Contact us for more information |
Assets | Streamline borrower experiences by verifying assets, including account balances, transaction histories and account holder identity information. This product has to be enabled separately in Development and Production. Contact us for more information |
Investments | Gain insight into a user’s investment accounts, including account balances, holdings, and transactions. This product has to be enabled separately in Development and Production. Contact us for more information |
Liabilities | Access liabilities data for student loans and credit cards. This product has to be enabled separately in Development and Production. Contact us for more information |
Institution coverage
As we noted above, an Item
represents accounts at a given financial institution, bank, or credit union. Plaid supports thousands of different institutions, and we’re constantly working to add more. Though integration information does change, Link stays up-to-date with Plaid’s latest institution coverage at all times and makes it easy for users to find their intended institution.
Because product coverage does vary by institution, we recommend initializing Link with all the products that your integration will need.